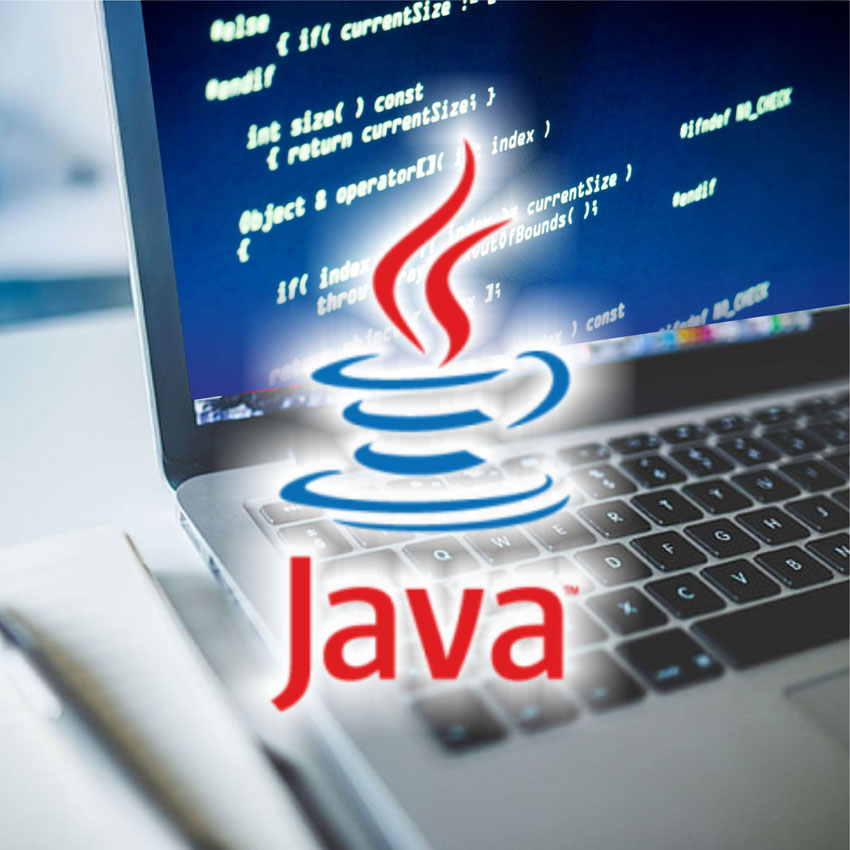
- 52 students
- 122 lessons
- 90 day duration
COURSE DESCRIPTION
Java is one of the world’s most important and widely used computer languages, and it has held this distinction for many years. Unlike some other computer languages whose influence has weared with passage of time, while Java’s has grown.
Java is a high level, robust, object-oriented and a secure and stable programming language but it is not a pure object-oriented language because it supports primitive data types like int, char etc.
Java is a platform-independent language because it has runtime environment i.e JRE and API. Here platform means a hardware or software environment in which anapplication runs.
Java codes are compiled into byte code or machine-independent code. This byte code is run on JVM (Java Virtual Machine).
The syntax is Java is almost the same as C/C++. But java does not support low-level programming functions like pointers. The codes in Java is always written in the form of Classes and objects.
As of 2020, Java is one of the most popular programming languages in use, especially for client-server web applications.Its has been estimated that there are around nine million Java developers inside the world.
-
Introduction
- Programming language Types and Paradigms.
- Computer Programming Hierarchy.
- How Computer Architecture Affects a Language ?
- Why Java ?
- Flavors of Java.
- Java Designing Goal.
- Role of Java Programmer in Industry.
- Features of Java Language.
- JVM –The heart of Java
- Java’s Magic Bytecode
- Language Fundamentals
-
The Java Environment:
- Installing Java.
- Java Program Development
- Java Source File Structure
- Compilation
- Executions.
-
Basic Language Elements:
- Lexical Tokens, Identifiers
- Keywords, Literals, Comments
- Primitive Datatypes, Operators
- Assignments
-
Object Oriented Programming
- Class Fundamentals.
- Object & Object reference.
- Object Life time & Garbage Collection.
- Creating and Operating Objects.
- Constructor & initialization code block.
- Access Control, Modifiers, methods
- Nested , Inner Class &Anonymous Classes
- Abstract Class & Interfaces
- Defining Methods, Argument Passing Mechanism
- Method Overloading, Recursion.
- Dealing with Static Members. Finalize() Method.
- Native Method. Use of “this “ reference.
- Use of Modifiers with Classes & Methods.
- Design of Accessors and Mutator Methods
- Cloning Objects, shallow and deep cloning
- Generic Class Types
-
Extending Classes and Inheritance
- Use and Benefits of Inheritance in OOP
- Types of Inheritance in Java
- Inheriting Data Members and Methods
- Role of Constructors in inheritance
- Overriding Super Class Methods.
- Use of “super”.
- Polymorphism in inheritance.
- Type Compatibility and Conversion
- Implementing interfaces.
-
Package
- Organizing Classes and Interfaces in Packages.
- Package as Access Protection
- Defining Package.
- CLASSPATH Setting for Packages.
- Making JAR Files for Library Packages
- Import and Static Import
- Naming Convention For Packages
-
Exception Handling:
- The Idea behind Exception
- Exceptions & Errors
- Types of Exception
- Control Flow In Exceptions
- JVM reaction to Exceptions
- Use of try, catch, finally, throw, throws in Exception Handling.
- In-built and User Defined Exceptions
- Checked and Un-Checked Exceptions
-
Array & String :
- Defining an Array
- Initializing & Accessing Array
- Multi –Dimensional Array
- Operation on String
- Mutable & Immutable String
- Using Collection Bases Loop for String
- Tokenizing a String
- Creating Strings using StringBuffer
-
Thread
- Understanding Threads
- Needs of Multi-Threaded Programming.
- Thread Life-Cycle
- Thread Priorities
- Synchronizing Threads
- Inter Communication of Threads
- Critical Factor in Thread -DeadLock
-
Applet
- Applet & Application
- Applet Architecture.
- Parameters to Applet
- Embedding Applets in Web page.
- Applet Security Policies
-
A Collection of Useful Classes
- Utility Methods for Arrays
- Observable and Observer Objects
- Date & Times
- Using Scanner
- Regular Expression
- Input/Output Operation in Java(java.io Package)
- Streams and the new I/O Capabilities
- Understanding Streams
- The Classes for Input and Output
- The Standard Streams
- Working with File Object
- File I/O Basics
- Reading and Writing to Files
- Buffer and Buffer Management
- Read/Write Operations with File Channel
- Serializing Objects
-
GUI Programming
- Designing Graphical User Interfaces in Java
- Components and Containers
- Basics of Components
- Using Containers
- Layout Managers
- Swing Componets
- Adding a Menu to Window
- Extending GUI Features Using Swing Components
-
Event Handling
- Event-Driven Programming in Java
- Event- Handling Process
- Event-Handling Mechanism
- The Delegation Model of Event Handling
- Event Classes
- Event Sources
- Event Listeners
- Avoiding Deadlocks in GUI Code
- Event Types & Classes
-
Networking Programming
- Networking Basics
- Client-Server Architecture
- Socket Overview
- Networking Classes and Interfaces
- Network Protocols
- Developing Networking Applications in Java
-
Database Programming using JDBC
- Introduction to JDBC
- JDBC Drivers & Architecture
- CURD operation Using JDBC
OUR METHODOLOGY
-
- 70% practical based and 30% theory-based.
- Version Control System like GIT (Git Hub) right from the beginnings, assignments/hands-on projects in-between the course to enhance the practical knowledge.
- Testing Framework like Unittest is a part of course to write TestCases for the application.
- Complete description contents in the form of PDF/PPT for every topic.
- Homework, assignments, and projects to teach the practical implementation of every theoretical topic.
- Practical sessions for every single topic so that students can understand how to manage applications using the version control systems and how to test and develop applications in real life for IT Industries.